Download a YouTube video with one line of Perl
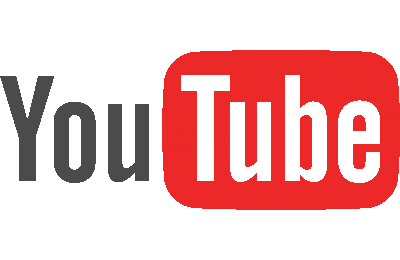
Downloading YouTube videos with Perl is easy when you’re packing the right module. That module is WWW::YouTube::Download. Here’s how you can download a video in one line of Perl.
Edit - this article was updated on 27/1/2014 to describe the “youtube-download” app that comes with WWW::YouTube::Download.
Warning
You should only download videos which you have permission to do so. The following is just an example of how to do this, when you have permission.
Requirements
You’ll need to install WWW::YouTube::Download. The CPAN Testers results for the latest version (0.56 at the time of writing) show that it runs on all major platforms.
You can install the module via CPAN at the command line:
$ cpan WWW:YouTube::Download
Use the youtube-download app
When you install WWW::YouTube::Download, it comes with a command-line app, “youtube-download”. Using it couldn’t be easier. Simply open the command line and type the program name with the URL or video id of the video to download. For example:
$ youtube-download http://www.youtube.com/watch?v=ju1IMxGSuNE
NB - using the app as shown above is the easiest way to use the tool - however at the time of writing I was not aware of the command line tool. Read on for the one liner example.
Download a video in one line of Perl
At the command line, type or paste the following command, replacing $id with the id of the video you want to download:
$ perl -MWWW::YouTube::Download -e 'WWW::YouTube::Download->new->download(q/$id/)'
Explaining the one liner
This one liner is simple. First we load WWW::YouTube::Download using the “-M” switch. Then the “-e” switch tells Perl to execute the code between the apostrophes. We then initiate a WWW::YouTube::Download object with new, and immediately call the download method on the new object. We use the quoting construct “q//” to quote strings without using quote marks as this makes the one liner more cross-platform compatible.
On Windows
On Windows, you’ll need to replace the apostrophes with double quotes (").
How to get the video id
The video id is the alphanumeric code value for “v” in the URL of the video you want to download. For example with this URL:
http://www.youtube.com/watch?v=ju1IMxGSuNE
“ju1IMxGSuNE” is the video id, because if you look in the URL after the question mark, v=ju1IMxGSuNE, which means “the value for v equals ju1IMxGSuNE”. If you have a URL but can’t work out the video id, WWW::YouTube::Download provides a video_id method. This one liner will print out the video id, just replace $url with the actual YouTube URL.
$ perl -MWWW::YouTube::Download -E 'say WWW::YouTube::Download->new->video_id(q{$url})'
A Perl script to download YouTube videos
We can expand the concepts used in the one liner into a fully-fledged Perl script, called “download.pl”:
#!/usr/bin/env perl
use strict;
use warnings;
use WWW::YouTube::Download;
if (@ARGV) {
my $tube = WWW::YouTube::Download->new;
my $video_id = $tube->video_id($ARGV[0]);
$tube->download($video_id, { filename => '{title}{suffix}' });
}
The script takes a YouTube URL as an argument. It gets the video id of the URL, then downloads the video into the current directory. As an added bonus, the script will save the file with the title of the video, instead of the default which is the video id. You can run the script at the command line, passing the URL of the YouTube video to download. For example:
$ ./download.pl http://www.youtube.com/watch?v=ju1IMxGSuNE
You may need to set the script’s permissions to executable using chmod:
$ chmod 755 download.pl
Conclusion
WWW::YouTube::Download is easy to use, fast and just works. The module’s documentation is easy to follow. Thanks to Yuji Shimada for writing it!
There is more to WWW::YouTube::Download than shown here - one interesting feature is that you can specify the video format (if more than one is available). By default the module downloads the highest quality video available.
This article was originally posted on PerlTricks.com.
Tags
David Farrell
David is a professional programmer who regularly tweets and blogs about code and the art of programming.
Browse their articles
Feedback
Something wrong with this article? Help us out by opening an issue or pull request on GitHub